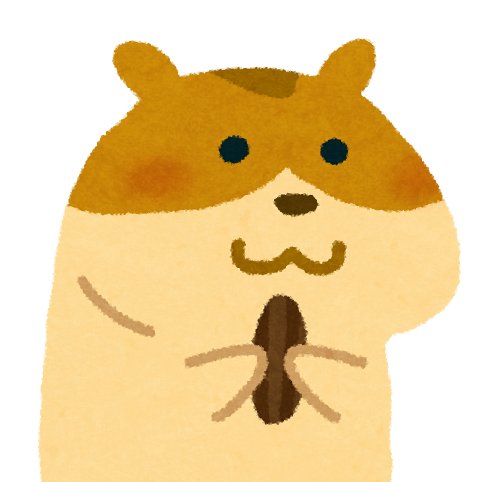
ショコラ
PHP PayPalでAPIを使って返金処理するには?
PayPal の返金処理をするサンプルプログラムです。
cURL ではなく file_get_contents を使用しています。
マニュアルに出てくる PayPalRequestId はなんでもいいみたいです。
元ネタは↓こちらです。
https://developer.paypal.com/docs/multiparty/issue-refund/

もっさん先輩
ペイパルで返金するプログラム
<?php
define('CLIENT_ID','{ペイパルの管理画面のクライアントID}');
define('APP_SECRET','{ペイパルの管理画面のシークレット}');
define('base','https://api-m.sandbox.paypal.com');
define('SELLER_PAYER_ID','{販売者のメールアドレス}');
$capture_id = "{取引ID}";
$amount = "{返金額}";
// APIリクエストの一意の識別子として使用されます。
// このヘッダーは、PayPalのサーバーに送信する各リクエストに一意のIDを付与するために使われ、
// トラッキングやデバッグの目的で便利です。
function generateUUID() {
return sprintf(
'%04x%04x-%04x-%04x-%04x-%04x%04x%04x',
mt_rand(0,0xffff),
mt_rand(0,0xffff),
mt_rand(0,0xffff),
mt_rand(0,0x0fff) | 0x4000,
mt_rand(0,0x3fff) | 0x8000,
mt_rand(0,0xffff),
mt_rand(0,0xffff),
mt_rand(0,0xffff)
);
}
function base64url($data) {
return rtrim(strtr(base64_encode($data),'+/','-_'),'=');
}
function getAuthAssertionValue() {
$header = array(
'alg' => 'none'
);
$encodedHeader = base64url(json_encode($header));
$payload = array(
'iss' => CLIENT_ID,
'payer_id' => SELLER_PAYER_ID,
);
$encodedPayload = base64url(json_encode($payload));
return $encodedHeader.'.'.$encodedPayload.'.';
}
function generateAccessToken() {
$url = base.'/v1/oauth2/token';
$auth = base64_encode(CLIENT_ID.':'.APP_SECRET);
$header = array(
"Authorization: Basic {$auth}",
'Accept: application/json',
'Accept-Language: en_US',
);
$context = array(
'http' => array(
'method' => 'POST',
'header' => implode("\r\n",$header),
'content' => 'grant_type=client_credentials',
'timeout' => 60, // second
),
);
$json = file_get_contents($url,false,stream_context_create($context));
return json_decode($json)?->access_token;
}
$url = base."/v2/payments/captures/{$capture_id}/refund";
$accessToken = generateAccessToken();
$pay_pal_request_id = generateUUID();
$jwt = getAuthAssertionValue();
$header = [
'Content-Type: application/json',
"Authorization: Bearer {$accessToken}",
"PayPal-Request-Id: {$pay_pal_request_id}",
"PayPal-Auth-Assertion: {$jwt}",
];
$context = [
'http' => [
'method' => 'POST',
'header' => implode("\r\n",$header),
'content' => '{"amount":{"value":"'.$amount.'","currency_code":"JPY"}}',
'timeout' => 60, // second
],
];
var_dump($context);
$json = file_get_contents($url,false,stream_context_create($context));
var_dump($json);
※sandbox用です。
以上