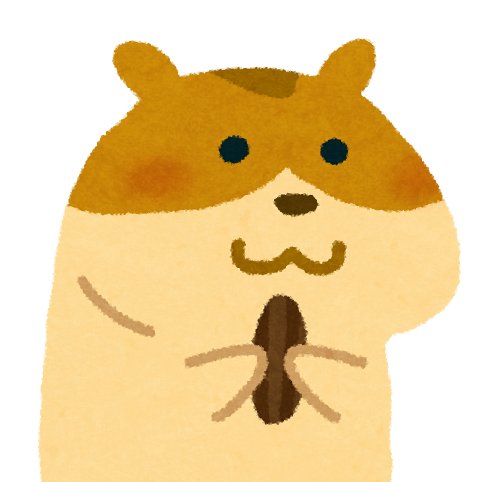
ショコラ
住所から緯度・経度・郵便番号を取得するには?
商用利用を考えているので、Google の Geocoding API にチャンレンジしてみます。
APIの有効化はこちら
https://console.cloud.google.com/apis/library/geocoding-backend.googleapis.com

もっさん先輩
PHPのプログラムだとこんな感じで緯度、経度、郵便番号を取得できます。
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class GoogleGeoCoding extends Command
{
protected $signature = 'geocoding';
protected $description = 'GoogleGeoCodingAPI のサンプル';
public function handle() {
$apiKey = env('GEMINI_API_KEY');
$client = new \Google_Client();
$client->setDeveloperKey($apiKey); // ←特に意味はない
// ジオコーディングしたい住所
$address = '東京都千代田区千代田1-1';
// 住所をURLエンコード
$address = urlencode($address);
// Geocoding APIのURLを作成
$url = "https://maps.googleapis.com/maps/api/geocode/json?address={$address}&key={$apiKey}";
// APIリクエストを送信
$httpClient = $client->getHttpClient();
$response = $httpClient->get($url);
// レスポンスをJSON形式でデコード
$data = json_decode($response->getBody(), true);
if ($data['status'] == 'OK') {
// 緯度・経度を取得
$latitude = $data['results'][0]['geometry']['location']['lat'];
$longitude = $data['results'][0]['geometry']['location']['lng'];
foreach ($data['results'][0]['address_components'] as $adrs ) {
if (in_array('postal_code',$adrs['types'])) {
$postal_code = str_replace('-','',$adrs['short_name']);
}
}
// 結果を表示
echo "緯度: {$latitude}\n";
echo "経度: {$longitude}\n";
echo "郵便番号: {$postal_code}\n";
}
else {
// エラーメッセージ
echo "ジオコーディングに失敗しました。\n";
echo "エラーメッセージ: " . $data['status'] . "\n";
}
}
}
上のプログラムは Google_Client を使えないかな~と考えたプログラムでして、使わない場合は↓のようになります。
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class GoogleGeoCoding2 extends Command
{
protected $signature = 'geocoding2';
protected $description = 'GoogleGeoCodingAPI のサンプル';
public function handle() {
$apiKey = env('GEMINI_API_KEY');
// ジオコーディングしたい住所
$address = '東京都千代田区千代田1-1';
// 住所をURLエンコード
$address = urlencode($address);
// Geocoding APIのURLを作成
$url = "https://maps.googleapis.com/maps/api/geocode/json?address={$address}&key={$apiKey}";
// APIリクエストを送信
$response = file_get_contents($url);
// レスポンスをJSON形式でデコード
$data = json_decode($response, true);
if ($data['status'] == 'OK') {
// 緯度・経度を取得
$latitude = $data['results'][0]['geometry']['location']['lat'];
$longitude = $data['results'][0]['geometry']['location']['lng'];
foreach ($data['results'][0]['address_components'] as $adrs ) {
if (in_array('postal_code',$adrs['types'])) {
$postal_code = str_replace('-','',$adrs['short_name']);
}
}
// 結果を表示
echo "緯度: {$latitude}\n";
echo "経度: {$longitude}\n";
echo "郵便番号: {$postal_code}\n";
}
else {
// エラーメッセージ
echo "ジオコーディングに失敗しました。\n";
echo "エラーメッセージ: " . $data['status'] . "\n";
}
}
}
以上