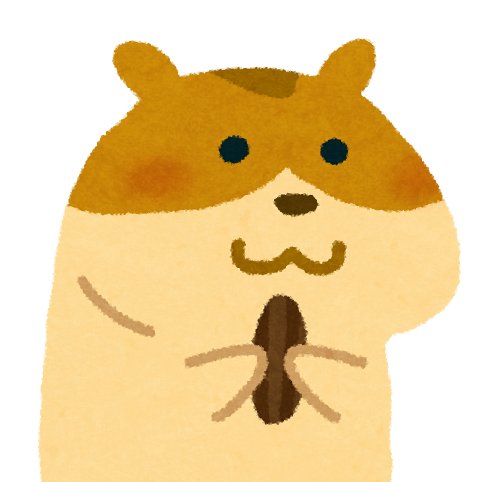
ショコラ
Laravel で Selenium を使ってみるには?
Laravel をデフォルトでインストールすると、Selenium も一緒にインストールされます。
きっと「アプリケーションのテストで使ってね」ということだと思いますが、今回は Selenium を使ってヤフーで検索してみようと思います。

もっさん先輩
手順
Laravel をインストールして Selenium を試す手順。
- プロジェクト名(selenium)を決めて以下のコマンドを実行します。
curl -s https://laravel.build/selenium | bash
インストール時にプロジェクト名のディレクトリが作成されます。
- インストールの最後に sudo でパスワードの入力を求められます。
↓下のメッセージが表示されてインストールは終わります。
Use 'docker scan' to run Snyk tests against images to find vulnerabilities and learn how to fix them
Get started with: cd selenium && ./vendor/bin/sail up
- sail のエイリアスを定義します。
echo "alias sail='[ -f sail ] && sh sail || sh vendor/bin/sail'" >> ~/.bashrc
source ~/.bashrc
Laravel のインストールはここまで。
- 「sail up」だと Ctrl+C でコンテナが止まってしまうので、「sail up -d」でコンテナを起動します。
cd selenium && ./vendor/bin/sail up -d
- PHP で Selenium を使うために、php-webdriver をインストールします。
sail composer require php-webdriver/webdriver
Selenium は コンテナで動作するので、他にインストールするものはありません。
- SeleniumTestコマンドを生成します。
sail artisan make:command SeleniumTest
生成されたファイルの中身です。app/Console/Commands/SeleniumTest.php
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
class SeleniumTest extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'command:name';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
return Command::SUCCESS;
}
}
- SeleniumTestコマンドを以下のように書き換えます。app/Console/Commands/SeleniumTest.php
<?php
namespace App\Console\Commands;
use Illuminate\Console\Command;
use Facebook\WebDriver\WebDriverBy;
use Facebook\WebDriver\WebDriverExpectedCondition;
use Facebook\WebDriver\Remote\RemoteWebDriver;
use Facebook\WebDriver\Remote\DesiredCapabilities;
class SeleniumTest extends Command
{
/**
* The name and signature of the console command.
*
* @var string
*/
protected $signature = 'selenium {keyword}';
/**
* The console command description.
*
* @var string
*/
protected $description = 'Command description';
/**
* Execute the console command.
*
* @return int
*/
public function handle()
{
$keyword = $this->argument('keyword');
// クロームを起動する。
$driver = RemoteWebDriver::create(
'http://selenium:4444/wd/hub',
DesiredCapabilities::chrome());
// ヤフーにアクセスする。
$driver->get('https://www.yahoo.co.jp');
// キーワードをクリックする。
$driver->findElement(WebDriverBy::name('p'))->click();
// キーワードを入力する。
$driver->getKeyboard()->sendKeys($keyword);
// 検索ボタンをクリックする。
$driver->findElement(WebDriverBy::cssSelector('button[type=submit]'))->click();
// 次のページのタイトルに、キーワードが含まれるまで待つ。
$driver->wait()->until(
WebDriverExpectedCondition::titleContains($keyword)
);
// 検索結果を表示する。
$html = $driver->findElement(WebDriverBy::xpath("//body"))->getText();
var_dump($html);
// クロームを終了する。
$driver->quit();
return Command::SUCCESS;
}
}
↑のポイントは「$driver->quit();」だと思います。quitして終わらないと、次回コマンドを実行すると固まってしまいます。
- selenium を使って検索してみましょう。
sail artisan selenium "laravel livewire"
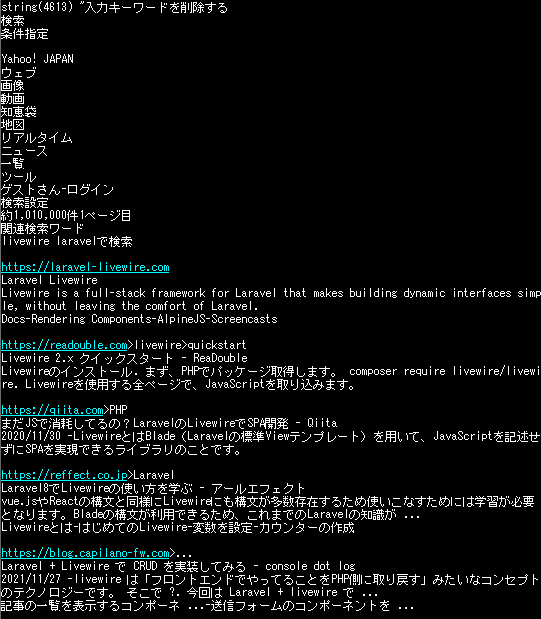
以上