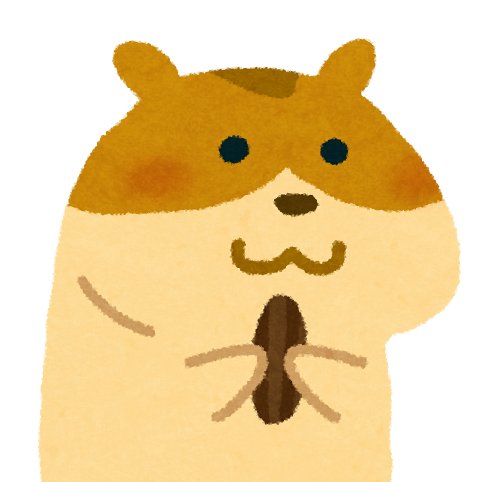
ショコラ
GraphQL について
GraphQL を使う案件がでてきましたので勉強です。
グラフィカルのホームページは https://graphql.org/ です。

もっさん先輩
GraphQL のはじめの一歩
- graphql をインストールします。
mkdir graphql
cd graphql
npm install express express-graphql graphql
- サンプルプログラムを作成します。
const express = require('express')
const app = express()
const { graphqlHTTP } = require('express-graphql')
const { buildSchema } = require('graphql')
app.use('/',graphqlHTTP({
// スキーマ定義
schema: buildSchema(`
type Query {
test: String
}
`),
// リゾルバ(実装)
rootValue:{
test:() => 'Hello Answorz!'
},
// GUIを使う。
graphiql: true,
})).listen(80) // 空いているポートを指定してください。
Error: listen EACCES: permission denied 0.0.0.0:80
↑のエラーが表示された場合は、別のアプリケーションでポート80が使われていますので、他のポートに変更してください。
- graphqlサーバーを起動します。
node server.js
- URL にアクセスします。
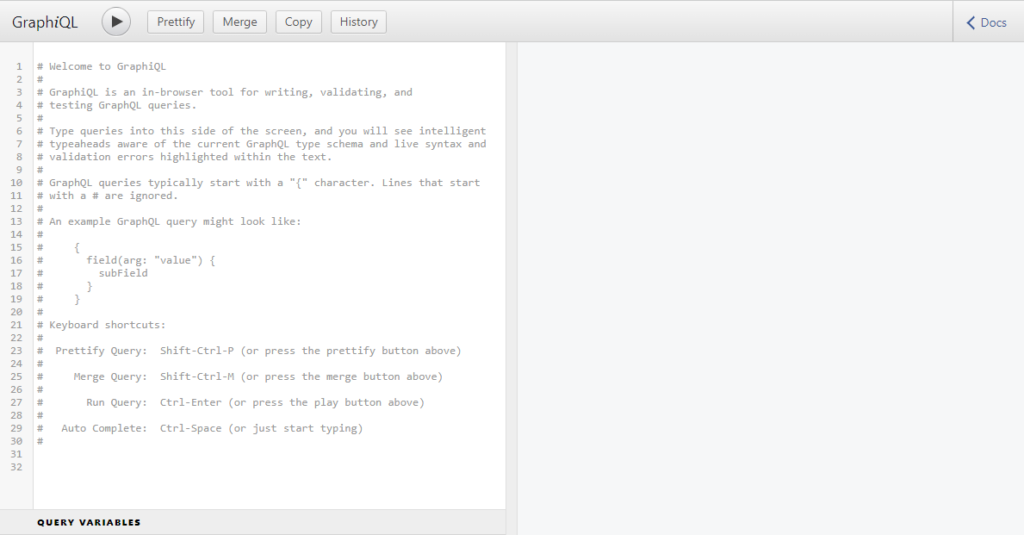
- 問い合わせます。
query {
test
}
test の結果が JSON で返ってきました。
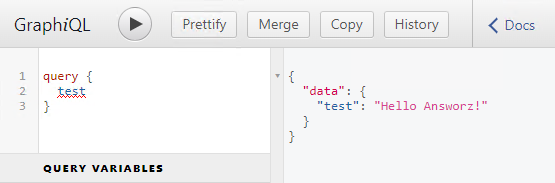
配列を使ってみる。
const express = require('express')
const app = express()
const { graphqlHTTP } = require('express-graphql')
const { buildSchema } = require('graphql')
app.use('/',graphqlHTTP({
// スキーマ定義
schema: buildSchema(`
type Query {
test:[String]
}
`),
// リゾルバ(実装)
rootValue:{
test:() => ['Hello Answorz1!','Hello Answorz2!']
},
// IDEを使う。
graphiql: true,
})).listen(80) // 空いているポートを指定してください。
問い合わせ方は↓こちらです。
{
test
}
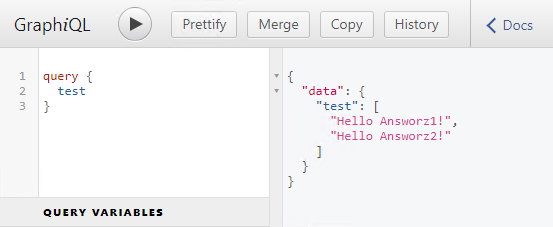
パラメーターを使ってみる。
const express = require('express')
const app = express()
const { graphqlHTTP } = require('express-graphql')
const { buildSchema } = require('graphql')
app.use('/',graphqlHTTP({
// スキーマ定義
schema: buildSchema(`
type Query {
test(ct:Int!):[Int]
}
`),
// リゾルバ(実装)
rootValue: {
test:({ct}) => {
let result = []
for (let i=0;i < ct;i++) {
result.push(Math.floor(Math.random() * 100))
}
return result
}
},
// IDEを使う。
graphiql: true,
})).listen(80) // 空いているポートを指定してください。
問い合わせ方は↓こちらです。
query {
test(ct:20)
}
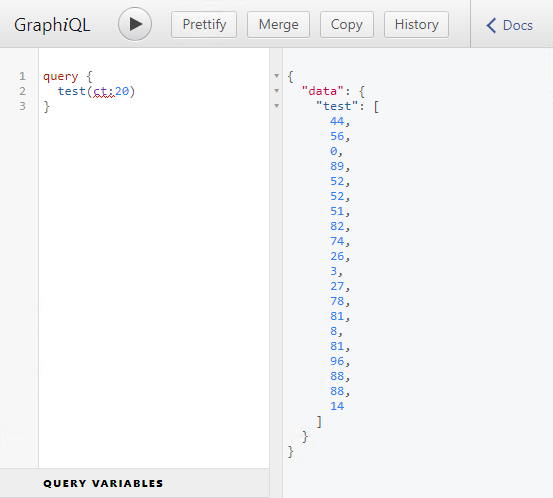
クラスを使ってみます。
class Person {
id
name
age
constructor(id,name,age) {
this.id = id
this.name = name
this.age = age
}
}
let db = {}
db[1] = new Person(1,'もっさん',40)
db[2] = new Person(2,'ショコラ',10)
const express = require('express')
const app = express()
const { graphqlHTTP } = require('express-graphql')
const { buildSchema } = require('graphql')
app.use('/',graphqlHTTP({
// スキーマ定義
schema: buildSchema(`
type Person {
id: Int
name: String
age: Int
}
type Query {
getPerson(id:Int!):Person
}
`),
// リゾルバ(実装)
rootValue: {
getPerson: ({id}) => db[id],
},
// IDEを使う。
graphiql: true,
})).listen(80) // 空いているポートを指定してください。
問い合わせをする場合、↓下のように取得するフィールドを指定します。カンマ必要無し。
query {
getPerson(id:2) {
id
name
age
}
}
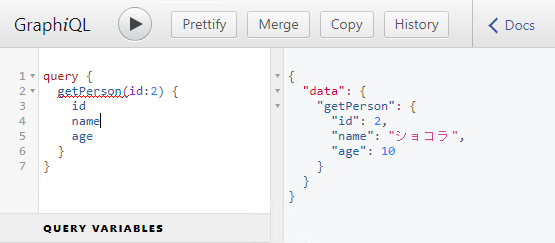
データを登録してみる。
この場合使うのが「type Mutation」です。
class Person {
id
name
age
constructor(id,name,age) {
this.id = id
this.name = name
this.age = age
}
}
let db = {}
const express = require('express')
const app = express()
const { graphqlHTTP } = require('express-graphql')
const { buildSchema } = require('graphql')
app.use('/',graphqlHTTP({
// リゾルバ(実装)
schema: buildSchema(`
type Person {
id:ID
name:String
age:Int
}
type Query {
getPerson(id:ID!):Person
}
type Mutation {
createPerson(id:ID!,name:String!,age:Int!): Person
updatePerson(id:ID!,name:String!,age:Int!): Person
}
`),
// 実装
rootValue:{
getPerson:({id}) => db[id],
createPerson:({id,name,age}) => db[id] = new Person(id,name,age),
updatePerson:({id,name,age}) => db[id] = new Person(id,name,age),
},
// IDEを使う。
graphiql: true,
})).listen(80) // 空いているポートを指定してください。
Mutation で登録します。
mutation {
createPerson(id:1,name:"もっさん",age:40) {
id
name
age
}
}
mutation {
createPerson(id:2,name:"ショコラ",age:10) {
id
name
age
}
}
問い合わせをしてみます。
query {
getPerson(id:1) {
id
name
age
}
}
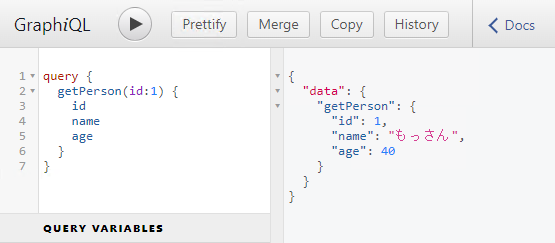
query {
getPerson(id:2) {
id
name
}
}
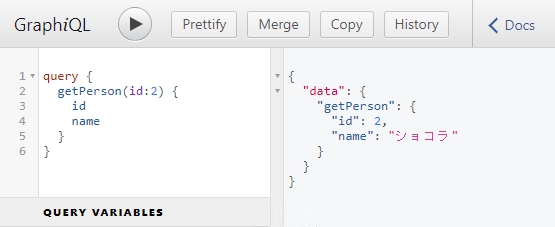
データをクラスで登録してみる。
↓クラスでデータを登録するとき定義で使うのが input。PersonInput に注目です。
class Person {
id
name
age
constructor(id,name,age) {
this.id = id
this.name = name
this.age = age
}
}
let db = {}
const express = require('express')
const app = express()
const { graphqlHTTP } = require('express-graphql')
const { buildSchema } = require('graphql')
app.use('/',graphqlHTTP({
// スキーマ定義
schema: buildSchema(`
type Person {
id:ID
name:String
age:Int
}
input PersonInput {
id:ID
name:String
age:Int
}
type Query {
getPerson(id:ID!):Person
}
type Mutation {
createPerson(input:PersonInput): Person
updatePerson(input:PersonInput): Person
}
`),
// リゾルバ(実装)
rootValue:{
getPerson:({id}) => db[id],
createPerson:({input}) => db[input.id] = input,
updatePerson:({input}) => db[input.id] = input,
},
// IDEを使う。
graphiql: true,
})).listen(80) // 空いているポートを指定してください。
Mutation で登録します。
mutation {
createPerson(input:{
id:1
name:"もっさん"
age:40
}) {
id
name
age
}
}
mutation {
createPerson(input:{
id:2
name:"ショコラ"
age:10
}) {
id
name
age
}
}
変数を使って登録してみます。
mutation ($input:PersonInput!) {
createPerson(input:$input) {
id
name
age
}
}
{
"input":{
"id":3,
"name":"ナイスガイ",
"age":20
}
}
今回は変数を使って問い合わせをしてみます。
query ($id:ID!) {
getPerson(id:$id) {
id
name
}
}
{"id":1}
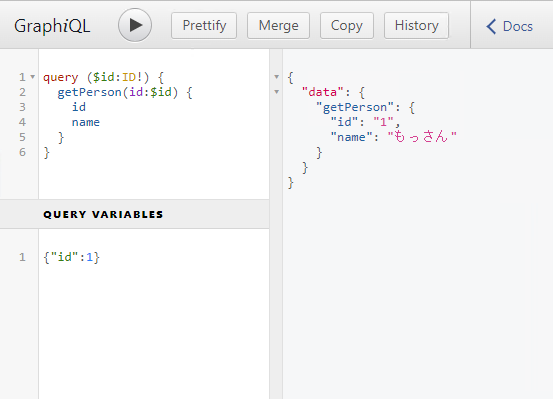
以上