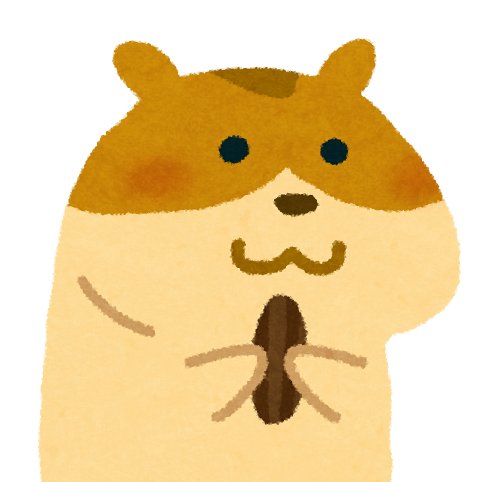
ショコラ
AI PHPで実装してみます
勉強したことをアウトプットしていきます。

もっさん先輩
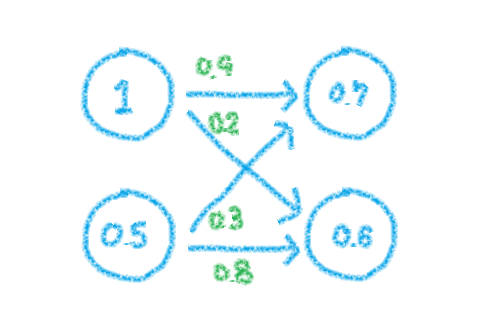
<?php
$input = [1.0,0.5];
$weight = [[0.9,0.2],[0.3,0.8]];
$output = [0,0];
function sigmoid( $x )
{
return 1 / (1 + exp(-1 * $x));
}
// 入力層の値にリンクの重みを掛けて出力層に保存します。
foreach ($input as $i => $in) {
foreach ($weight[$i] as $j => $w) {
$output[$j] += $in * $w;
}
}
// 出力層にシグモイド関数を適用します。
foreach ($output as $i => $o) {
$output[$i] = sigmoid($o);
}
var_dump($output);
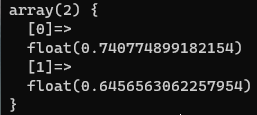
<?php
$input = [0.9,0.1,0.8];
$weight1 = [[0.9,0.2,0.1],[0.3,0.8,0.5],[0.4,0.2,0.6]];
$hidden = [0,0,0];
$weight2= [[0.3,0.6,0.8],[0.7,0.5,0.1],[0.5,0.2,0.9]];
$output = [0,0,0];
function sigmoid( $x )
{
return 1 / (1 + exp(-1 * $x));
}
// 入力層にリンクの重みを掛けて隠れ層に保存する。
foreach ($input as $i => $in) {
foreach ($weight1 [$i] as $j => $w) {
$hidden[$j] += $in* $w;
}
}
// 隠れ層にシグモイド関数を適用する。
foreach ($hidden as $i => $v) {
$hidden[$i] = sigmoid($v);
}
var_dump($hidden);
// 隠れ層にリンクの重みを掛けて出力層に保存する。
foreach ($hidden as $i => $h) {
foreach ($weight2[$i] as $j => $w) {
$output[$j] += $h * $w;
}
}
// 出力層にシグモイド関数を適用する。
foreach ($output as $i => $o) {
$output[$i] = sigmoid($o);
}
var_dump($output);
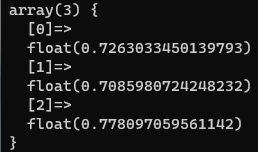
<?php
$error = [0.8,0.5];
$weight2 = [[2.0,1.0],[3.0,4.0]];
$hidden = [0,0];
// 誤差を逆伝播する。(出力層→隠れそう)
foreach ($error as $i => $e) { //$e = 0.8
$total = 0;
foreach ($weight2 as $w) {
$total += $w[$i];
}
foreach ($weight2 as $j => $w) {
$hidden[$j] += $e* $w[$i] / $total;
}
}
var_dump($hidden);
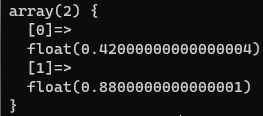
<?php
$error = [0.8,0.5];
$weight2 = [[2.0,1.0],[3.0,4.0]];
$weight1 = [[3.0,1.0],[2.0,7.0]];
$hidden = [0,0];
$input = [0,0];
// 誤差を逆伝播する。(出力層→隠れそう)
foreach ($error as $i => $e) { //$e = 0.8
$total = 0;
foreach ($weight2 as $w) {
$total += $w[$i];
}
foreach ($weight2 as $j => $w) {
$hidden[$j] += $e* $w[$i] / $total;
}
}
// 誤差を逆伝播する。(隠れそう→入力層)
foreach ($hidden as $i => $e) { //$e = 0.8
$total = 0;
foreach ($weight1 as $w) {
$total += $w[$i];
}
foreach ($weight1 as $j => $w) {
$input[$j] += $e* $w[$i] / $total;
}
}
var_dump($input);
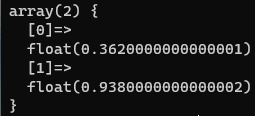
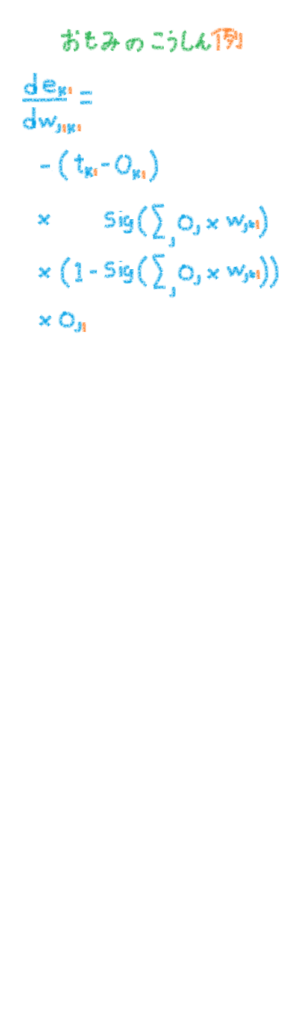
リンクの重みを更新する処理
<?php
$error = [0.8,0.5];
$weight2 = [[2.0,1.0],[3.0,4.0]];
$hidden = [0.4,0.5];
function sigmoid( $x )
{
return 1 / (1 + exp(-1 * $x));
}
foreach ($error as $i => $e)
{
$total = 0;
foreach ($weight2 as $j => $w) {
$total += $w[$i] * $hidden[$j];
}
$sigm = sigmoid($total);
foreach ($weight2 as $j => $w) {
$d = -1 * ($e) * $sigm * (1 - $sigm) * $hidden[$j];
$weight2[$i][$j] += -0.1 * $d;
}
}
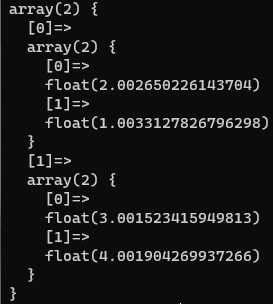
以上